The Ying Part of Yang
As a human living in the 21st century, you have probably watched a few movies here and there. There are specific things that should be familiar. One of them is Ying-Yang. That famous Chinese symbol that signifies:
- The good in evil
- The evil in good
That being said, drawing Ying-Yang with a graphics package shouldn’t take long, provided you know how to draw circles and fill them. But then, can you algorithmically think about the process? The purpose of the whole courses on Turtle Graphics is to make you think in algorithms. So, let’s get started. The first example I’ll show is an inefficient algorithm I designed myself. It’s long, procedural but simple. The second is that which is part of the Turtle Graphics demo. It’s short, efficient, quite clear and shows the adeptness of its writer.
My Algorithm
It all starts with importing the module. Then using a function to define what’s involved. Finally, the function is called at the end of the code.
from turtle import *
def Ying(radius):
'this whole function block draws just one fish'
width(3) # the width of the pen. From 1 to 3.
circle(radius/2, 180) # a circle with half the defined radius and a half arc ( essentially the fish's head
circle(radius, 180)
right(-180) # turn the pen's head anticlockwise by 180 degrees
circle(-radius/2, 180) # the smaller body circle
right(-90)
penup()
forward(radius/1.3)
pendown()
right(-90)
circle(radius/4)
right(-90)
penup()
forward(radius/1.3)
pendown()
right(90)
Ying(120) # the first fish
Ying(120) # the second fish
This code only draws the fishes without filling them with colour (this will be shown in the next code). Colour will be added using a neat trick.
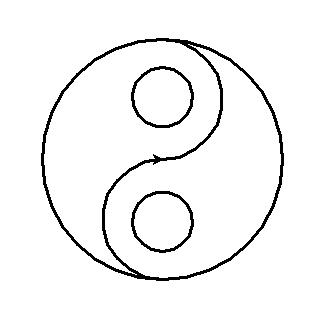
this is the current result. Run on IDLE.
from turtle import *
def Ying(radius, color1, color2):
'this whole function block draws just one fish'
width(3) # the width of the pen. From 1 to 3.
color("black", color1) # now, this defines the color of the fish's body
begin_fill()
circle(radius/2, 180) # a circle with half the defined radius and a half arc (essentially the fish's head)
circle(radius, 180)
left(180) # turn the pen's head anticlockwise by 180 degrees
circle(-radius/2, 180) # the smaller body circle
end_fill()
color(color1, color2) # this defines that of its eyes
begin_fill()
right(-90)
penup()
forward(radius/1.3)
pendown()
right(-90)
circle(radius/4)
end_fill()
right(-90)
penup()
forward(radius/1.3)
pendown()
right(90)
Ying(120, "black", "white") # the first fish
Ying(120, "white", "black") # the second fish
Now that should produce this:

The purpose of showing the first is to encourage you. It took me quite a while to produce that code, even though I had help from the original code. Thinking in algorithms takes quite a while. This may be caused by our brains doing most mental tasks in the background. algorithmic thinking requires active thinking.
The original code
Here’s the turtle demo algorithm. You can compare it with mine.
""" turtle-example-suite:
tdemo_yinyang.py
Another drawing suitable as a beginner's
programming example.
The small circles are drawn by the circle
command.
"""
from turtle import *
def yin(radius, color1, color2):
width(3)
color("black", color1)
begin_fill()
circle(radius/2., 180)
circle(radius, 180)
left(180)
circle(-radius/2., 180)
end_fill()
left(90)
up()
forward(radius*0.35)
right(90)
down()
color(color1, color2)
begin_fill()
circle(radius*0.15)
end_fill()
left(90)
up()
backward(radius*0.35)
down()
left(90)
def main():
reset()
yin(200, "black", "white")
yin(200, "white", "black")
ht()
return "Done!"
if __name__ == '__main__':
main()
mainloop()
I hope you grabbed a thing or two. Review the code and modify it anyway you like. It’s highly encouraged. Just remember this: DFTBA!