WOW! Coloured Peace?
Peace is something humans all wish for. It’s a topic that everyone talks about. But then, the formula for perfect peace has never been discovered.
There’s a particular symbol that represents peace. Personally, I view it as the unity of four hands. We will be reproducing this formula with python turtle graphics today.
The code
from turtle import *
def main():
colors =( "red3", "orange", "yellow",
"seagreen4", "orchid4",
"royalblue1", "dodgerblue4") # so, these are colors that'll be drawn
pu()
goto(-300, -190) # to start somewhere at the base
pd()
for Individualitem in colors: # a for loop that iterates through the colors list
color(Individualitem)
width(40)
fd(600)
pu()
bk(600)
lt(90)
fd(40)
rt(90)
pd()
color('white') #this is the start of the peace symbol
width(15)
pu()
setposition(0, -190) # the drawing starts from the base
pd()
circle(120)
lt(90)
fd(240)
goto(0, -190)
x = clone() # a quite intersting function. It clones the current turtle.
y = clone()
x.fd(130) # but then, the clobne has to be controlled like any other turtle
x.lt(135)
x.fd(125)
y.fd(130)
y.rt(135)
y.fd(125)
hideturtle()
x.hideturtle()
y.hideturtle()
if __name__ == "__main__":
'''this block initializes the main function that has previously been defined'''
main()
mainloop() # and this enables the window to run continously
If you are currently using pydroid, you can make the following modification.
- change the goto function on line 7 to
goto(-200, -190)
2. Change the forward and backward distance (line 14 and 16) to 400. It could be 450, depending on your screen size.
fd(400)
pu()
bk(400)
You could ignore all this, and use your landscape mode. That’ll solve all issues.
Extra Explanation
- The python program starts with importing the turtle module (all of it).
- Then the main function (method) is defined. This is more professional than using a procedural approach
- The colours used are enclosed in a tuple. Remember that tuples are iterables just like strings, lists and dictionaries
- Then the pen is taken to the bottom region of the screen. The turtle starts from the middle (0, 0) by default.
- A for loop is then used to iterate through the tuple, then the commands under the for loop are executed.
- The turtle goes forward with the pen down
- It then goes backwards with the pen up
- Then its head moves towards the left direction
- a forward movement is executed
- The angle return back to the initial
- The next peacecolor is then used
- The turtle takes new properties and draws a circle starting from the base
- It then draws a straight line from bottom up.
- At this point, two clones are produced named x and y. These clones are like mirror siblings. I’ll use x to explain
- The clone moves forward
- It then turns towards the left by 135 degrees
- It then moves forward again to draw the small “arm”
The output
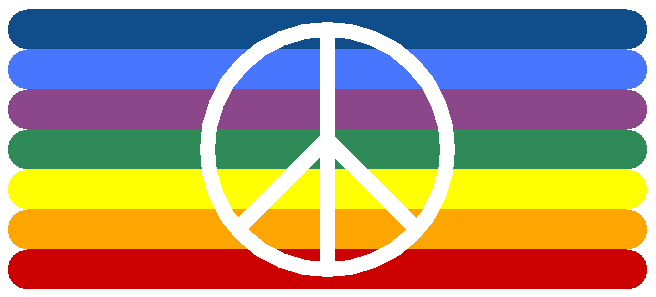
Conclusion
This code is quite explanatory. But you could always follow the extra instructions given. No complex algorithm has been used yet in the course of this journey, but we will encounter more beautiful and challenging examples. Just remember DFTBA!